用js 写一个 图片可以双指放大缩小单指移动的程序
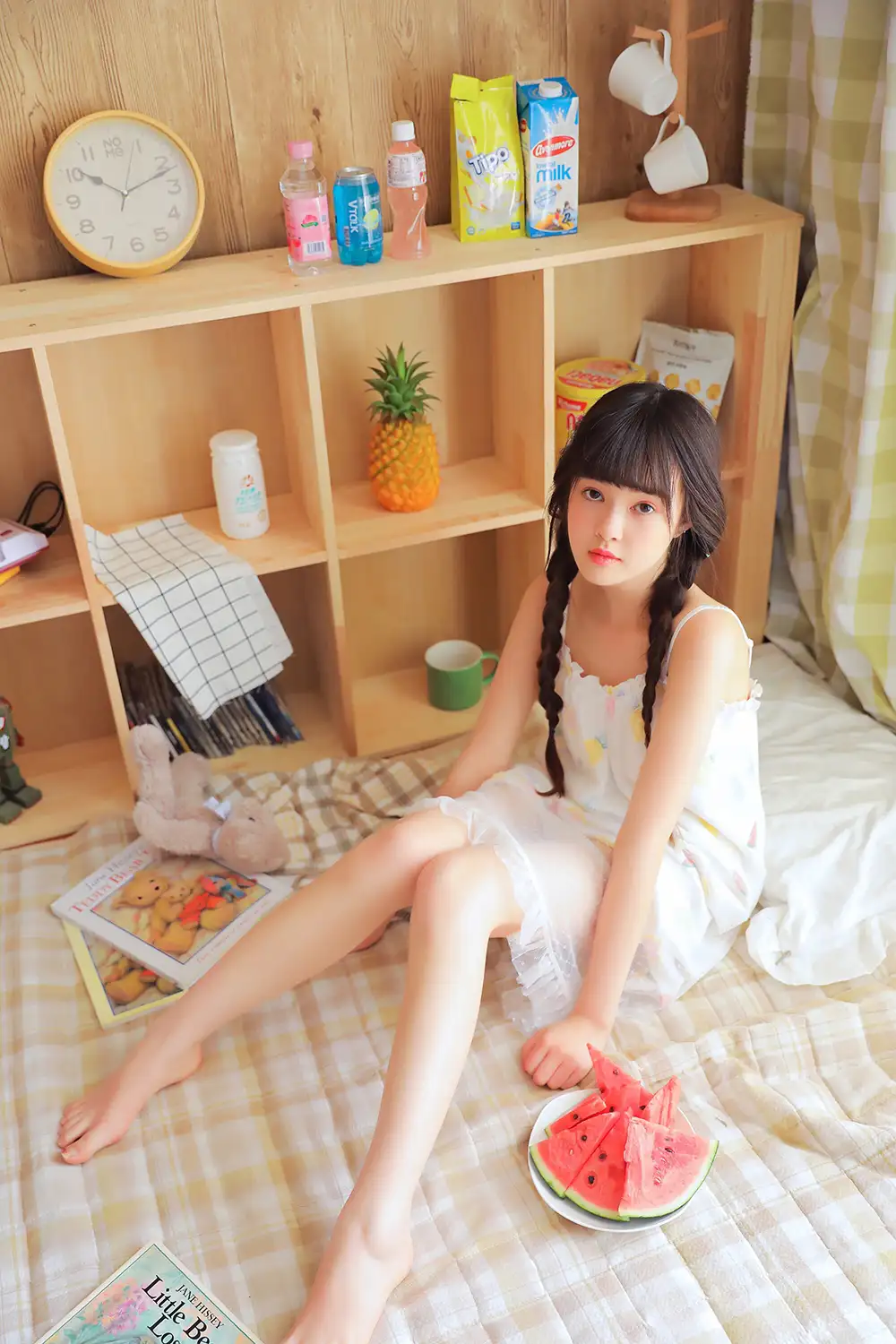
以下是使用JavaScript编写的一个简单的程序,可以实现图片的双指放大缩小和单指移动功能:
<!DOCTYPE html>
<html>
<head>
<style>
#image-container {
position: relative;
width: 500px;
height: 500px;
overflow: hidden;
}
#image-container img {
position: absolute;
width: 100%;
height: 100%;
cursor: move;
}
</style>
</head>
<body>
<div id="image-container">
<img src="your-image.jpg" alt="Image">
</div>
<script>
window.onload = function() {
var imageContainer = document.getElementById('image-container');
var image = imageContainer.getElementsByTagName('img')[0];
var initTouchDistance = 0;
var initImageWidth = 0;
imageContainer.addEventListener('touchstart', function(e) {
e.preventDefault();
var touches = e.touches;
if (touches.length === 2) { // 双指操作
initTouchDistance = Math.sqrt(
Math.pow(touches[0].pageX - touches[1].pageX, 2) +
Math.pow(touches[0].pageY - touches[1].pageY, 2)
);
initImageWidth = image.offsetWidth;
}
});
imageContainer.addEventListener('touchmove', function(e) {
e.preventDefault();
var touches = e.touches;
if (touches.length === 2) { // 双指操作
var currentTouchDistance = Math.sqrt(
Math.pow(touches[0].pageX - touches[1].pageX, 2) +
Math.pow(touches[0].pageY - touches[1].pageY, 2)
);
var scale = currentTouchDistance / initTouchDistance;
image.style.width = initImageWidth * scale + 'px';
} else if (touches.length === 1) { // 单指操作
var touch = touches[0];
var left = touch.pageX - imageContainer.offsetLeft;
var top = touch.pageY - imageContainer.offsetTop;
image.style.left = left + 'px';
image.style.top = top + 'px';
}
});
};
</script>
</body>
</html>
上述代码中,通过触摸事件实现了图片的双指放大缩小和单指移动功能。通过监听touchstart
事件获取初始的双指距离和图片宽度,然后在touchmove
事件中计算当前的双指距离与初始距离的比例,并根据比例调整图片的宽度实现放大缩小效果。同时,也通过touchmove
事件监听单指操作,根据单指的位置调整图片的位置实现移动效果。
原文地址: https://www.cveoy.top/t/topic/jg0W 著作权归作者所有。请勿转载和采集!